Friday, March 11, 2011, 12:24 AM - Web
I finally solved one of the issues my Pic a Day gallery had with Internet Explorer:For any reason, IE (tryed on IE6, IE7, IE8) is unable to dynamically create a not yet declared variable when you directly affect the result of some function.
Javascript is supposed to automatically create global scope variable if you affect something into an undeclared variable:
foo = 5;
...will instanciate the global "foo" variable if not yet declared.
However, if you assign the result of some functions to an unexisting variable, IE simply fails and stops evaluating the funtion where the issue occurs:
foo = document.getElementById('bar');
Assuming there is a element called 'bar', then this line fails if 'foo' is not yet declared (but this works as expected under Firefox 3.x). Declaring 'foo' before using it solves this Internet Explorer issue:
var foo;
foo = document.getElementById('bar');
| 0 trackbacks
| permalink
| related link
Sunday, September 14, 2008, 06:47 PM - Web
Having a line of text which is as wide as its container width, sound simple? Well, actually it is not that easy.For my pic a day gallery I wanted to put a navigation interface under the thumbnails, and to have this navigation interface extend to the full possible width. Of course, as the width changes according to the browser window, it can not be specified in pixels.
A possibility would be to use a single row table, and to put each navigation element within an individual table cell, but first it is far from an elegant solution, second I did not thought about this possibility when doing the navigation interface.
As the navigation interface is made from text elements, I thought about simply using the text-align: justify CSS property. However, this doesn't justify the last line of a paragraph, and in my case the navigation interface is a single line. CSS3 is supposed to address this issue, by the text-align-last property, which allows to set justification method for the last line of a paragraph. Problem: it is not yet supported by browsers.
So here is the trick: using Javascript to increase spacing between words, until it fills the whole container width.
First, set a small words spacing, then iteratively increase it. While doing this, check the element height. When the height increase, it means that your text was wrapped over a new line. You can then roll back words by one step, and you are done: your line now fills the whole width:
function expandNavigation() {
document.getElementById('config').style.opacity = 0;
navi = document.getElementById('navigation');
navi.style.opacity = 0;
var h0 = navi.scrollHeight;
var em = 0.2;
var em_inc = 0.2;
do {
em += em_inc;
navi.style.wordSpacing = em + 'em';
} while (navi.scrollHeight == h0)
em -= em_inc;
navi.style.wordSpacing = em + 'em';
fadeIn('navigation', 0, 15);
fadeIn('config', 0, 15);
}
In order to avoid flickering while the word spacing is increased, I also hid the text while iterating, and only made it visible again at when the optimal word spacing is found (the function fadeIn sets the text back to its visible state).
Tuesday, July 1, 2008, 08:57 PM - Web
By random browsing, I found a web optimization article about using sprites within CSS.Sprites are basically a set of small size graphics that were used a long time ago to simulate characters animation within video games. There was a predefined set of postures per character, and by alternating between them you could produce a "fake" animation.
Now, back on the CSS topic, the article explains how this old trick can be used to speed up web pages. It's obviously a hack, but quite an elegant one. Let's say you have a few arrows and icons you would want to display within a web page.
First, you put all of them within a single image, in this way:
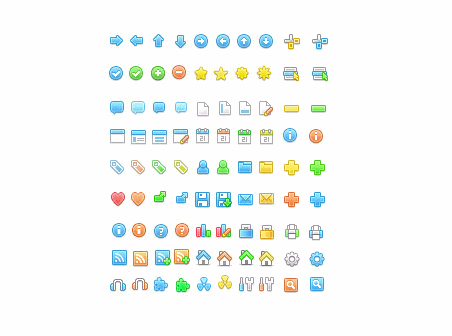
(icons courtesy of ineversay.com)
Then you set the background of some element of your web page (table cell, list,...) to your sprite image, and using a combination of positioning you correctly set up your element to use the correct sprite (by using the "background-position" CSS property).
What do you save this way?
*A bit of bandwidth related to the graphics, as combined graphics are using less space than the sum of the individual graphic files (mainly because of the headers that would be repeated within each file)
*Most important, you reduce the number of individual http requests. That reduces the needed bandwidth, but also reduces the load of the http server.
This trick can also be used neatly for rollovers, using a single graphic instead of separate ones. Positioning is then even easier, as you could simply alternate background-position from left to right
a:link, a:visited {
display: block;
width: 127px;
height:25px;
line-height: 25px;
color: #000;
text-decoration: none;
background: #fc0 url(image-rolloverdual.png) no-repeat left top;
text-indent: 25px;
}
a:hover {
/* background: #c00; */
background-position: right top;
color: #fff;
}